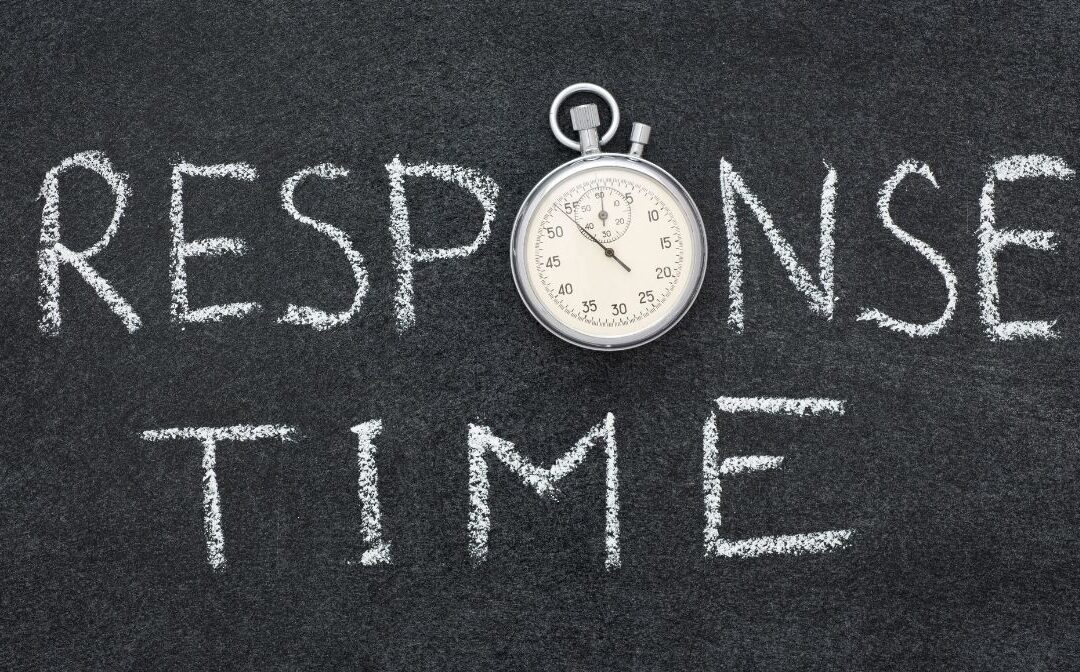
Improving Server Response Time: Essential Tips and Strategies for Optimal Performance
In the digital age, server response time is critical for providing a seamless user experience and maintaining high search engine rankings. A slow server can lead to frustrated users, increased bounce rates, and lost revenue. This guide covers essential strategies for improving server response time, focusing on selecting the right hosting plan, optimizing server performance, and leveraging Content Delivery Networks (CDNs) for faster global access.
Choosing the Right Hosting Plan for Performance
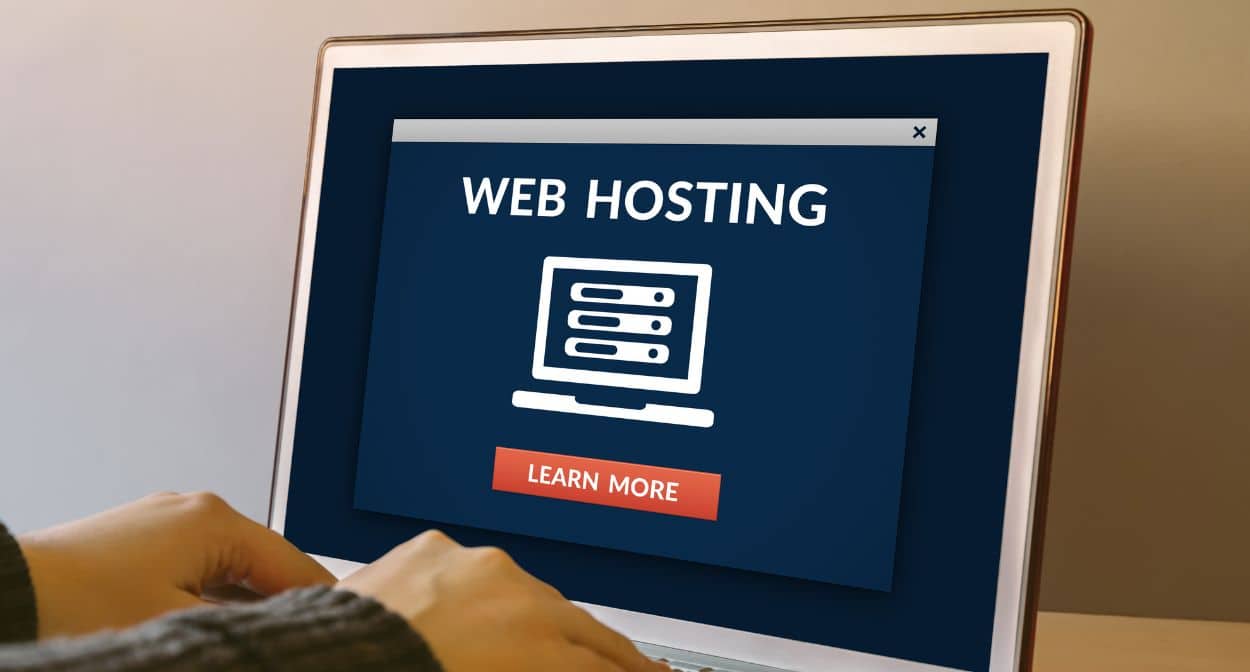
Shared Hosting
Shared hosting is the most affordable option, where multiple websites share the same server resources. While cost-effective, this plan can lead to slower response times during peak traffic periods. Shared hosting is suitable for small websites with low traffic but may not be ideal for growing businesses.
Virtual Private Server (VPS) Hosting
VPS hosting offers a middle ground between shared and dedicated hosting. It provides a virtualized environment with dedicated resources, leading to better performance and more control over server settings. VPS is a good option for medium-sized websites that require more stability and faster response times.
Dedicated Hosting
Dedicated hosting provides an entire server for your website, ensuring maximum performance and control. This option is ideal for large websites with high traffic volumes. Although more expensive, dedicated hosting offers superior server response times and enhanced security.
Cloud Hosting
Cloud hosting uses a network of virtual servers to host websites. This setup allows for scalable resources and high availability. Cloud hosting is an excellent choice for websites that experience fluctuating traffic, as it can dynamically adjust resources to maintain optimal performance.
Server Optimization Techniques
Once you have chosen the right hosting plan, several optimization techniques can further enhance server response times.
Upgrading to a Faster Server
One of the most straightforward ways to improve server response time is by upgrading to a faster server with more CPU, RAM, and storage capabilities. This upgrade ensures that your server can handle higher loads and process requests more quickly.
Optimizing Database Queries
Efficient database management is crucial for reducing server response times. Here are some tips for optimizing database queries:
- Indexing: Create indexes on frequently queried columns to speed up data retrieval.
- Query Optimization: Rewrite complex queries to be more efficient and use fewer resources.
- Database Caching: Implement caching mechanisms to store frequently accessed data in memory, reducing the need for repeated database queries.
- Regular Maintenance: Perform regular maintenance tasks such as defragmenting indexes and optimizing database tables to ensure optimal performance.
Caching
Caching stores copies of frequently accessed data to reduce server load and response times. There are several types of caching:
- Page Caching: Stores static versions of pages, reducing the need for dynamic content generation.
- Object Caching: Caches data objects, such as database results, to speed up data retrieval.
- Opcode Caching: Stores compiled PHP code to avoid recompilation on each request.
Content Delivery Networks (CDNs) for Faster Global Access
A Content Delivery Network (CDN) is a network of servers distributed across various geographic locations. CDNs cache and deliver content from the server closest to the user, significantly reducing latency and improving load times.
Benefits of Using a CDN
- Reduced Latency: By serving content from the nearest server, CDNs minimize the distance data travels, resulting in faster load times.
- Improved Reliability: CDNs distribute traffic across multiple servers, reducing the risk of downtime due to server overload.
- Scalability: CDNs can handle large volumes of traffic, ensuring consistent performance during traffic spikes.
- Enhanced Security: Many CDNs offer security features such as DDoS protection and SSL encryption, safeguarding your website from malicious attacks.
Implementing a CDN
To implement a CDN, follow these steps:
Choose a CDN Provider: Select a CDN provider that fits your needs and budget. Popular options include Cloudflare, Akamai, and Amazon CloudFront.
Configure Your CDN: Set up your CDN by linking it to your website’s DNS settings and configuring caching rules.
Test and Monitor: After implementation, test your website’s performance and monitor the CDN’s impact on load times and server response.
Conclusion
Improving server response time is crucial for providing a superior user experience and maintaining strong search engine rankings. By selecting the right hosting plan, optimizing server performance, and leveraging CDNs, you can significantly enhance your website’s speed and reliability. Implement these strategies to ensure your website performs optimally, regardless of traffic volume or geographic location.